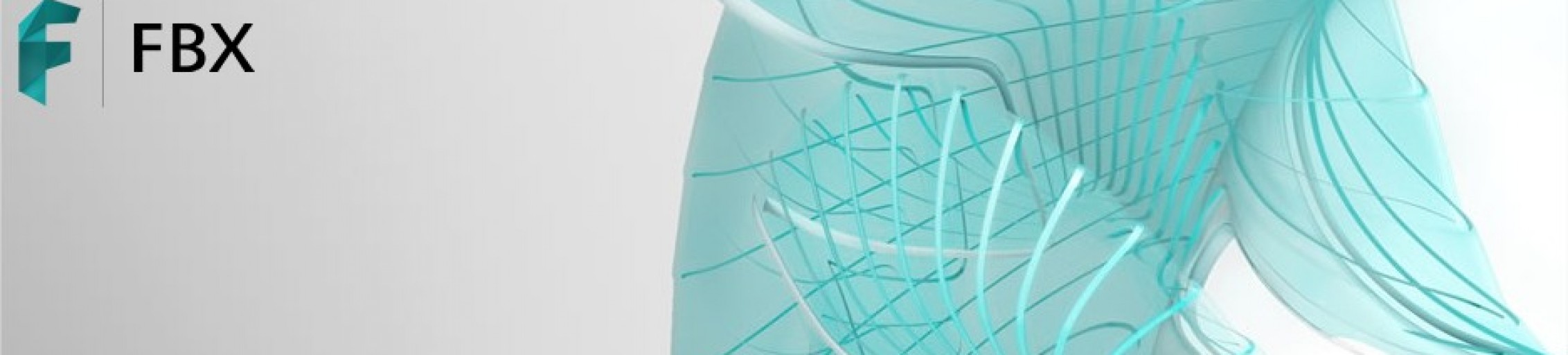
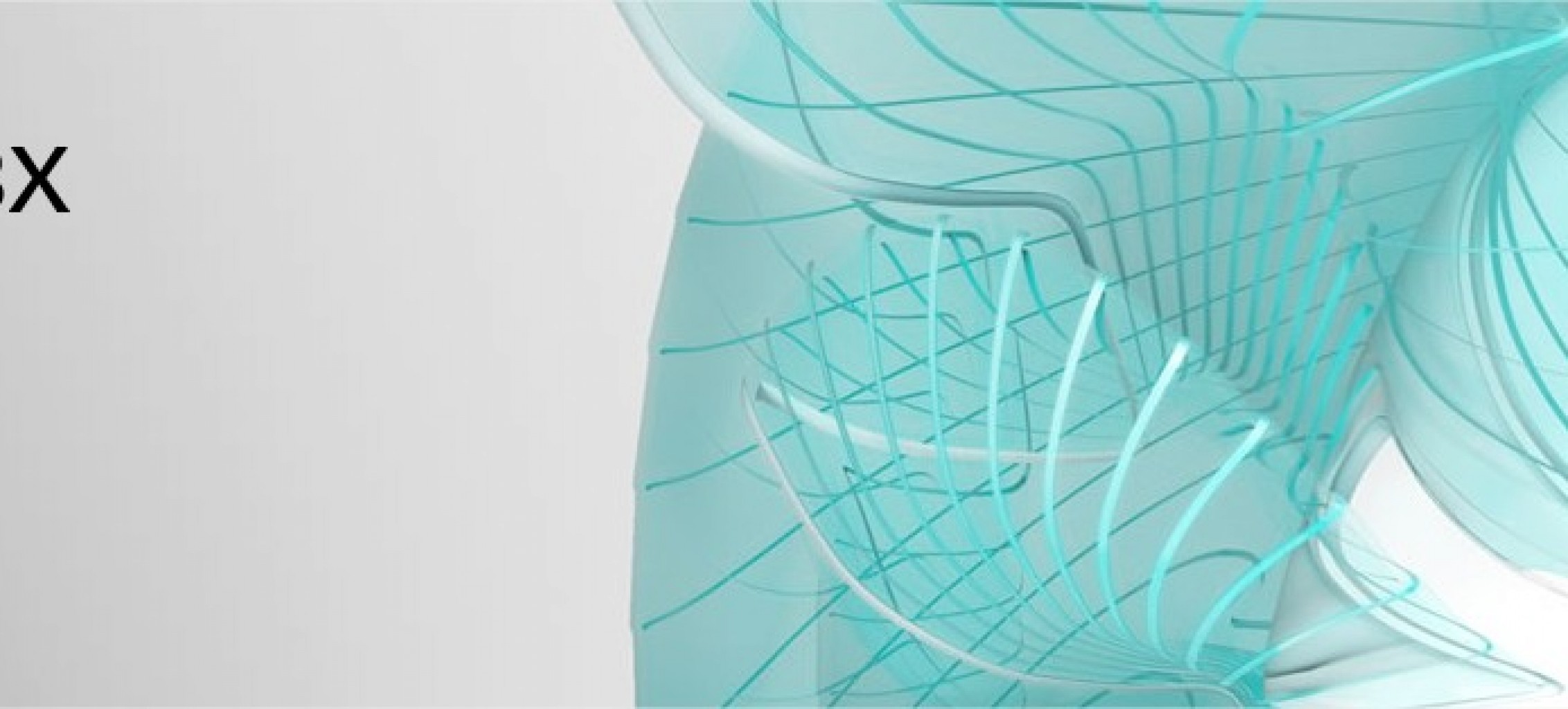
ArcaneManagedFbx
ArcaneManagedFbx
Archived
This is archived and is no longer being supported. Please refer to the source code licensing for more information on how you can extend or support it yourself. The project itself is incomplete, and requires additional work in order to be suitable for prod
This is an incomplete C# wrapper for the Autodesk FBX SDK that is written in C++.
The library was originally intended for creating MonoGame "Content Processor" for importing, processing, and rendering FBX assets. Initially only a limited subset of functions and classes were intended to be supported or made available through CLR and .NET.
Written in C++/CLI, the DLL is intended to be used for interfacing with the C++ Autodesk FBX SDK, which is a collection of static libraries that are intended for loaded FBX assets within a native application. The FBX file format is proprietary and supported by Autodesk.
Usage
Find below a (very old) sample code snippet of what can be done with it.
public void Load()
{
LogMessage("Instantiating the FBX Manager...");
FBXManager managerInstance = ArcManagedFBX.FBXManager.Create();
if (string.IsNullOrEmpty(Filename))
throw new FileNotFoundException("The file that is being loaded in does not exist!");
if (!File.Exists(Filename))
throw new FileNotFoundException("The file that is being loaded was not found on disk.");
LogMessage("Instantiating the FBX Settings...");
FBXIOSettings settings = FBXIOSettings.Create(managerInstance, "IOSRoot");
managerInstance.SetIOSettings(settings);
LogMessage("Loading plugins directory...");
managerInstance.LoadPluginsDirectory(Environment.CurrentDirectory, "");
int fileMajorNumber = 0, fileMinorNumber = 0, fileRevisionNumber = 0;
LogMessage("Instantiating the Scene...");
FBXScene scene = FBXScene.Create(managerInstance, "My Scene");
FBXImporter importer = FBXImporter.Create(managerInstance, "");
// Instantiate the framework for the scene as required.
m_SceneFramework = new SceneFramework(scene);
LogMessage("Load the importer for the file '{0}'",Filename);
bool initializeResult = importer.Initialize(Filename, -1, managerInstance.GetIOSettings());
FBXManager.GetFileFormatVersion(ref fileMajorNumber,ref fileMinorNumber,ref fileRevisionNumber);
bool importResult = importer.Import(scene);
m_SceneFramework.Operation();
if (importResult)
PerformTests(scene);
else
LogError("Import failed. Nothing to do!");
}
Relevant Links